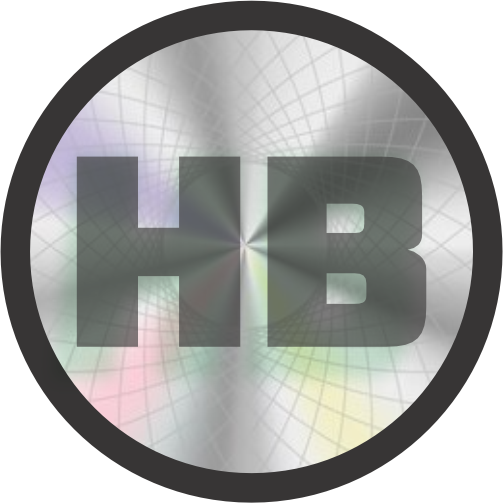
1. Introduction
Humanbot Pro™ is an advanced embeddable 3D chatbot solution designed to provide a human-like conversational experience directly on your website. It features a photorealistic 3D avatar with synchronized facial animations and natural speech capabilities, all while maintaining lightweight performance.
Core Features
Realistic 3D Avatar
Lifelike humanoid character with synchronized facial animations
Ultra-Lightweight
Only 5MB impact on your website
Natural Language Processing
AI-powered conversations using state-of-the-art LLMs
Multi-language Support
Built-in support for 72+ languages
Voice Integration
Advanced text-to-speech with ElevenLabs, Whisper, or Google TTS
Cross-browser Compatibility
Works seamlessly on all modern browsers
Responsive Design
Adapts perfectly to desktop and mobile interfaces
Secure Architecture
Backend-proxied API calls for credential protection
Product Differentiation
What sets Humanbot Pro apart from traditional chatbots:
- Human-like Interaction: The combination of 3D visualization, facial expressions, and voice creates a genuinely human-like experience.
- Ultra-Concise Responses: Designed for clarity and efficiency with brief, direct answers.
- Performance Optimization: Advanced resource management ensures minimal impact on website performance.
- Multi-modal Input/Output: Users can type or speak, and the assistant responds with synchronized voice and facial expressions.
- Enterprise-grade Reliability: Robust error handling, memory management, and cross-origin communication.
2. Technologies & Architecture
Humanbot Pro leverages cutting-edge web technologies to deliver its immersive experience:
Core Technologies
- Babylon.js (v5.0.0): High-performance 3D rendering engine
- WebGL: Hardware-accelerated graphics rendering
- Web Speech API: Native speech recognition capabilities
- ElevenLabs API: Premium multilingual text-to-speech synthesis
- Large Language Models: Integration with OpenAI, Anthropic, or Mistral
- ES6+ JavaScript: Modern JavaScript with optimal resource management
- Responsive CSS3: Adaptive layout with hardware-accelerated animations
Architecture Overview
┌─────────────────────────────────────┐
│ Client Side │
├─────────────┬───────────┬───────────┤
│ 3D Rendering│ Speech │ Chat UI │
│ (Babylon) │ Processing│ Components│
├─────────────┴───────────┴───────────┤
│ Resource Management │
├─────────────────────────────────────┤
│ Embedding System │
└───────────────┬─────────────────────┘
│
┌───────────────┴─────────────────────┐
│ Server Side │
├─────────────┬───────────────────────┤
│ API Proxy │ Configuration │
│ Services │ Management │
└─────────────┴───────────────────────┘
Key System Components
- Embedding System: Handles cross-origin communication, iframe management, and resource loading
- 3D Rendering Engine: Manages avatar visualization, animations, and performance optimization
- Facial Animation System: Controls lifelike facial expressions and lip synchronization
- Speech Processing: Handles voice input/output with fallback mechanisms
- Chat Handler: Manages conversation flow, context, and business logic
- Resource Manager: Optimizes memory usage, cleanup, and performance
- API Proxy Services: Securely communicates with external APIs (LLMs, TTS)
3. Getting Started
Installation
Standard Implementation
<script src="https://cdn.humanbot.pro/embed.min.js" async></script>
Advanced Implementation (with Configuration)
<script>
window.HUMANBOT_CONFIG = {
apiKey: 'YOUR_API_KEY',
model: 'anthropic', // Options: 'openai', 'anthropic', 'mistral'
language: 'en',
voiceEnabled: true,
autoOpen: false
};
</script>
<script src="https://cdn.humanbot.pro/embed.min.js" async></script>
NPM Installation (for React, Vue, Angular)
npm install @humanbot/pro
import { HumanbotPro } from '@humanbot/pro';
// Initialize
const chatbot = new HumanbotPro({
apiKey: 'YOUR_API_KEY',
// Other configuration options
});
// Open programmatically
chatbot.open();
Configuration
Humanbot Pro can be configured using the businesjs.json
file or by providing a configuration object during initialization.
Essential Configuration Parameters
Parameter | Type | Description |
---|---|---|
apiKey |
String | Your Humanbot Pro API key |
model |
String | LLM provider ('openai', 'anthropic', 'mistral') |
systemMessage |
String | Custom instructions for the AI assistant |
language |
String | Default language code (e.g., 'en', 'fr', 'bg') |
voiceEnabled |
Boolean | Enable/disable voice capabilities |
bookingSuggestions |
Array | Custom booking suggestion messages |
productHighlights |
Array | Key product features to highlight |
autoOpen |
Boolean | Automatically open chatbot on page load |
position |
String | Button position ('bottom-right', 'bottom-left', etc.) |
theme |
Object | Custom theme colors and styling |
Complete Configuration Example
{
"apiKey": "YOUR_API_KEY",
"model": "anthropic",
"systemMessage": "You are Sophia from Humanbot Pro. CRITICAL RULES: (1) ONLY use the pre-selected language (English, Bulgarian, or French). (2) Keep responses ULTRA-SHORT - maximum 1 sentence for information. (3) Sound casual and human, not like a chatbot.",
"language": "en",
"voiceEnabled": true,
"bookingSuggestions": {
"English": [
"Our site has demo booking if you're interested.",
"We do have demos available if you wanna check it out."
]
},
"productHighlights": {
"English": [
"Humanbot Pro is lightweight (only 5MB) and won't slow down your website.",
"We support 72+ languages with natural conversation capabilities."
]
},
"theme": {
"primary": "#088ccc",
"background": "rgba(28, 28, 28, 0.95)",
"text": "#ffffff"
},
"position": "bottom-right",
"autoOpen": false
}
4. Customization Options
Visual Customization
Humanbot Pro offers extensive visual customization options to match your brand:
Theme Colors
window.HUMANBOT_CONFIG = {
theme: {
primary: "#ff5500", // Primary accent color
secondary: "#333333", // Secondary color
background: "#ffffff", // Background color
text: "#000000", // Text color
buttonBackground: "#ff5500", // Button background
buttonText: "#ffffff" // Button text color
}
};
Button Customization
window.HUMANBOT_CONFIG = {
button: {
text: "Chat With Us", // Button text
icon: "https://yourdomain.com/custom-icon.png", // Custom icon
position: "bottom-left", // Position (bottom-right, bottom-left, etc.)
style: {
borderRadius: "10px", // Custom border radius
boxShadow: "none", // Custom shadow
padding: "10px 20px" // Custom padding
}
}
};
Avatar Customization
Premium plans include customization of the 3D avatar:
window.HUMANBOT_CONFIG = {
avatar: {
model: "custom", // Use custom model
modelUrl: "https://yourdomain.com/custom-avatar.glb", // URL to custom GLB file
scale: 1.2, // Size adjustment
position: { x: 0, y: 0, z: 0 }, // Position adjustment
animations: {
idle: "CustomIdle", // Custom animation names
talk: ["CustomTalk1", "CustomTalk2"]
}
}
};
Behavioral Customization
Conversation Style
window.HUMANBOT_CONFIG = {
personality: {
conversationStyle: "friendly", // Options: professional, friendly, casual
responseLength: "concise", // Options: concise, standard, detailed
formalityLevel: "casual", // Options: formal, neutral, casual
traits: {
empathetic: 0.8, // 0.0 to 1.0
assertive: 0.3, // 0.0 to 1.0
knowledgeable: 0.9 // 0.0 to 1.0
}
}
};
Business Logic
window.HUMANBOT_CONFIG = {
business: {
bookingFrequency: 3, // Suggest booking every X messages
prioritizeLeads: true, // Focus on lead generation
handoffThreshold: 5, // Transfer to human after X complex questions
workingHours: {
start: "09:00", // Office hours start
end: "17:00", // Office hours end
timezone: "UTC+2", // Office timezone
offHoursMessage: "We're currently closed. Leave a message and we'll get back to you."
}
}
};
5. Integration Systems
CRM Integration
Humanbot Pro provides seamless integration with popular CRM systems to capture leads, track conversations, and sync customer data.
Supported CRM Platforms
- Salesforce - Complete integration with leads, contacts, and opportunities
- HubSpot - Full contact and deal synchronization
- Zoho CRM - Lead capture and contact management
- Pipedrive - Deal creation and contact management
- Microsoft Dynamics - Enterprise-level integration
- Custom CRM - Integration via API endpoints
CRM Integration Configuration
window.HUMANBOT_CONFIG = {
integrations: {
crm: {
provider: "hubspot", // CRM provider name
apiKey: "YOUR_CRM_API_KEY", // CRM API key or token
portal: "YOUR_PORTAL_ID", // For HubSpot specifically
mappings: {
// Map chatbot properties to CRM fields
firstName: "contact.firstName",
lastName: "contact.lastName",
email: "contact.email",
company: "company.name",
phone: "contact.phone",
interest: "deal.description"
},
triggers: {
// Define when to create/update CRM records
createContact: "email_provided",
createDeal: "booking_requested",
updateContact: "conversation_end"
},
createTasks: true, // Create follow-up tasks
assignTo: "default_owner", // Assign to specific team member
dealPipeline: "default", // Specific pipeline for deals
dealStage: "initial_contact" // Initial stage for new deals
}
}
};
Lead Qualification
Configure advanced lead qualification rules to prioritize high-value prospects:
window.HUMANBOT_CONFIG = {
integrations: {
crm: {
// ... other CRM settings ...
leadQualification: {
enabled: true,
rules: [
// Qualification rules
{
field: "budget", // What metric to check
condition: "greaterThan", // Comparison type
value: 10000, // Threshold value
score: 30 // Points to add if true
},
{
field: "timeline",
condition: "lessThan",
value: 3, // Months
score: 20
},
{
field: "industry",
condition: "equals",
value: ["healthcare", "finance", "education"],
score: 15
}
],
thresholds: {
hot: 50, // Score needed for hot lead
warm: 30, // Score needed for warm lead
cold: 0 // Default for cold lead
},
autoAssign: {
hot: ["john@example.com", "sarah@example.com"],
warm: ["team@example.com"],
cold: "marketing@example.com"
}
}
}
}
};
Custom CRM Events
Define custom events to trigger specific CRM actions:
window.HUMANBOT_CONFIG = {
integrations: {
crm: {
// ... other CRM settings ...
customEvents: [
{
trigger: "demo_scheduled", // Event name
action: "createTask", // Action to take
data: {
type: "Follow-up Demo",
dueDate: "+2 days",
assignTo: "sales.rep",
priority: "high"
}
},
{
trigger: "pricing_requested",
action: "updateContact",
data: {
status: "Sales Qualified Lead",
interest: "Pricing Information",
lastActivity: "now"
}
}
]
}
}
};
JavaScript API for CRM Integration
Programmatically interact with the CRM integration:
// Log a custom CRM event
window.humanbotPro.logCrmEvent('pricing_requested', {
productTier: 'enterprise',
expectedUsers: 500
});
// Create a CRM contact directly
window.humanbotPro.createCrmContact({
firstName: 'John',
lastName: 'Doe',
email: 'john.doe@example.com',
company: 'Acme Inc',
phone: '+1234567890'
});
// Update CRM record with conversation data
window.humanbotPro.updateCrmRecord('contact', 'john.doe@example.com', {
lastConversation: new Date().toISOString(),
interestLevel: 'high',
notes: 'Interested in enterprise deployment'
});
Real-time Database Integration
Humanbot Pro can connect to real-time database services to store conversation state, user information, and analytics data.
Supported Real-time Database Platforms
- Firebase Realtime Database - Full integration with Google's real-time solution
- Supabase - PostgreSQL with real-time capabilities
- MongoDB Realm - Document-based real-time sync
- AWS AppSync - GraphQL-based real-time data sync
- Custom WebSocket - Integration with custom real-time backends
Firebase Realtime Database Configuration
window.HUMANBOT_CONFIG = {
integrations: {
realtime: {
provider: "firebase",
config: {
apiKey: "YOUR_FIREBASE_API_KEY",
authDomain: "your-app.firebaseapp.com",
databaseURL: "https://your-app.firebaseio.com",
projectId: "your-app",
storageBucket: "your-app.appspot.com",
messagingSenderId: "123456789",
appId: "1:123456789:web:abc123def456"
},
collections: {
conversations: "conversations",
users: "users",
analytics: "chatbot_analytics"
},
syncOptions: {
syncConversationHistory: true,
syncUserProfiles: true,
syncIntents: true,
syncMetrics: true
},
securityRules: {
userScope: true, // Limit data access to user
encryptSensitiveData: true, // Encrypt PII data
retentionPeriod: 90 // Days to keep data
}
}
}
};
Real-time Conversation State
Configure persistent conversation state across sessions:
window.HUMANBOT_CONFIG = {
integrations: {
realtime: {
// ... other realtime settings ...
conversationState: {
persistent: true, // Remember conversations
expiryDays: 30, // How long to remember
encryptedFields: ["email", "phone", "address"],
structure: {
messages: true, // Store message history
context: true, // Store conversation context
userProfile: true, // Store user information
intents: true, // Store detected intents
entities: true // Store extracted entities
},
indexedFields: [
"userProfile.email",
"intents.primary",
"entities.product"
]
}
}
}
};
Querying Real-time Data
Access and query real-time data programmatically:
// Get all conversations for a user
window.humanbotPro.realtime.getConversations(userId)
.then(conversations => {
console.log('User conversations:', conversations);
});
// Listen for new messages in real-time
window.humanbotPro.realtime.subscribeToMessages(conversationId, (newMessage) => {
console.log('New message received:', newMessage);
});
// Query conversations with specific intent
window.humanbotPro.realtime.queryConversations({
field: 'intents.primary',
operator: '==',
value: 'pricing_inquiry'
}).then(results => {
console.log('Pricing inquiries:', results);
});
// Update user profile in real-time
window.humanbotPro.realtime.updateUserProfile(userId, {
lastVisit: new Date().toISOString(),
pageViews: increment(1),
interests: arrayUnion('new-feature')
});
Advanced Real-time Analytics
Track and analyze conversation metrics in real-time:
window.HUMANBOT_CONFIG = {
integrations: {
realtime: {
// ... other realtime settings ...
analytics: {
enabled: true,
metrics: {
// Standard metrics
messageCount: true,
responseTime: true,
sessionDuration: true,
intentDetection: true,
// Advanced metrics
sentimentAnalysis: true,
topicModeling: true,
confusionMatrix: true,
engagementScore: true
},
dimensions: {
// Group by these dimensions
timeOfDay: true,
dayOfWeek: true,
userSegment: true,
intentCategory: true,
referralSource: true
},
events: {
// Track these custom events
bookingRequested: true,
productViewed: true,
feedbackGiven: true,
handoffRequested: true
}
}
}
}
};
Webhook Integration System
Humanbot Pro provides a powerful webhook system to integrate with external services and create automation workflows.
Webhook Configuration
window.HUMANBOT_CONFIG = {
integrations: {
webhooks: {
endpoints: [
{
name: "newLeadWebhook",
url: "https://your-service.com/api/new-lead",
events: ["conversation_started", "email_provided", "phone_provided"],
headers: {
"Authorization": "Bearer YOUR_API_KEY",
"Content-Type": "application/json"
},
method: "POST",
retryCount: 3,
retryDelay: 1000
},
{
name: "slackNotification",
url: "https://hooks.slack.com/services/YOUR_SLACK_WEBHOOK",
events: ["high_priority_query", "negative_sentiment", "human_handoff"],
method: "POST",
transformPayload: true,
payloadTemplate: {
"text": "New {{event}} detected",
"blocks": [
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "*New {{event}}*\nUser: {{user.email}}\nQuery: {{lastMessage}}"
}
}
]
}
}
],
globalSettings: {
sendConversationContext: true,
includeTimestamp: true,
includeUserAgent: true,
includePageUrl: true,
signatureSecret: "YOUR_WEBHOOK_SECRET"
}
}
}
};
Custom Webhook Events
Define and trigger custom webhook events:
window.HUMANBOT_CONFIG = {
integrations: {
webhooks: {
// ... other webhook settings ...
customEvents: [
{
name: "pricing_plan_selected",
trigger: "intent",
intentName: "select_pricing_plan",
extractFields: {
planName: "entities.pricing_plan",
userCount: "entities.user_count",
billingCycle: "entities.billing_cycle"
}
},
{
name: "high_value_prospect",
trigger: "condition",
conditions: [
{
field: "entities.budget",
operator: "greaterThan",
value: 10000
},
{
field: "entities.company_size",
operator: "greaterThan",
value: 100
}
],
combineOperator: "AND"
}
]
}
}
};
Trigger Webhooks Programmatically
// Trigger a custom webhook event
window.humanbotPro.triggerWebhook('pricing_plan_selected', {
planName: 'Enterprise',
userCount: 500,
billingCycle: 'annual',
discount: '20%'
});
// Add global webhook data available to all webhook calls
window.humanbotPro.setWebhookGlobalData({
campaignSource: 'spring_promotion',
abTestVariant: 'pricing_variant_b',
affiliateId: 'partner123'
});
// Get status of webhook delivery
window.humanbotPro.getWebhookStatus('newLeadWebhook')
.then(status => {
console.log('Webhook status:', status);
// { success: true, lastDelivery: '2023-04-12T15:30:22Z', errorCount: 0 }
});
6. Advanced Configuration
Base Models Configuration
Humanbot Pro allows for deeply customized AI base models that can be configured to answer all business-specific questions accurately.
Model Selection and Configuration
window.HUMANBOT_CONFIG = {
ai: {
provider: "anthropic", // AI provider: 'openai', 'anthropic', 'mistral'
model: "claude-3-haiku", // Base model name
apiVersion: "2023-06-01", // API version
temperature: 0.7, // Response randomness (0.0-1.0)
contextWindow: 16000, // Max tokens in context window
maxTokens: 1024, // Max response tokens
topP: 0.9, // Nucleus sampling
presencePenalty: 0.6, // Penalize repetition
frequencyPenalty: 0.2, // Penalize frequent tokens
instructionFormat: "system", // System message format for instructions
fallbackProvider: "openai", // Fallback if primary fails
debugging: false // Enable debugging logs
}
};
Knowledge Base Integration
Create a comprehensive knowledge base for your business:
window.HUMANBOT_CONFIG = {
knowledgeBase: {
enabled: true,
sources: [
// Source documents
{
name: "product_documentation",
type: "url",
location: "https://docs.yourcompany.com/api/",
updateFrequency: "weekly",
priority: 0.9
},
{
name: "pricing_info",
type: "json",
data: [
{
"plan": "Basic",
"price": "$29/month",
"features": ["Feature A", "Feature B", "Feature C"],
"userLimit": 10
},
{
"plan": "Pro",
"price": "$99/month",
"features": ["Feature A", "Feature B", "Feature C", "Feature D", "Feature E"],
"userLimit": 50
},
{
"plan": "Enterprise",
"price": "Custom",
"features": ["All Pro Features", "Feature F", "Feature G", "Feature H"],
"userLimit": "Unlimited"
}
],
priority: 1.0
},
{
name: "faq_database",
type: "structured",
data: [
{
"question": "What payment methods do you accept?",
"answer": "We accept all major credit cards, PayPal, and bank transfers for annual plans."
},
{
"question": "How do I cancel my subscription?",
"answer": "You can cancel anytime from your account dashboard under 'Subscription'. Refunds are processed according to our refund policy."
},
{
"question": "Is there a free trial?",
"answer": "Yes, we offer a 14-day free trial with full access to all features. No credit card required."
}
],
priority: 0.8
}
],
settings: {
retrievalStrategy: "hybrid", // vector + keyword search
vectorizationModel: "all-mpnet-base-v2",
maxResults: 5, // Top results to use
retrievalThreshold: 0.75, // Minimum relevance score
cacheResults: true, // Cache retrievals
cacheDuration: 86400, // 24 hours
rewriteQuery: true, // Rewrite user query for better retrieval
filterByIntent: true // Use detected intent to filter results
}
}
};
Custom Business Entities
Define business-specific entities for improved understanding:
window.HUMANBOT_CONFIG = {
entities: {
// Custom entity definitions
products: [
{
"id": "prod_001",
"name": "SocialBoost Pro",
"category": "Marketing",
"price": 299,
"features": ["Scheduled Posting", "Analytics Dashboard", "Competitor Analysis"],
"targetAudience": ["Small Business", "Marketing Teams"],
"testimonials": [
{ "name": "John Smith", "company": "Acme Inc", "quote": "Increased our engagement by 240%" }
],
"faqs": [
{ "question": "Does it work with Instagram?", "answer": "Yes, fully integrated with Instagram." }
]
},
// More product entities...
],
services: [
{
"id": "serv_001",
"name": "Implementation Support",
"description": "Full white-glove setup and configuration",
"price": "Custom",
"duration": "2-4 weeks",
"deliverables": ["System setup", "Team training", "Custom integrations"],
"process": ["Discovery call", "Requirements gathering", "Implementation", "Testing", "Handover"]
},
// More service entities...
],
team: [
{
"id": "team_001",
"name": "Sarah Johnson",
"title": "Account Manager",
"expertise": ["Enterprise Accounts", "Healthcare Industry", "Integration Planning"],
"availability": "Monday-Friday, 9am-5pm EST",
"contactMethod": "Booking required via dashboard"
},
// More team entities...
],
// Additional entity types...
}
};
Ultra-Advanced Super JSON Configuration
The following complete example shows a comprehensive model configuration with all business logic elements:
{
"ai": {
"provider": "anthropic",
"model": "claude-3-opus",
"temperature": 0.5
},
"systemMessage": "You are Sophia, a helpful and knowledgeable representative for TechCorp Solutions. You specialize in our cloud computing services, with deep expertise in our product offerings, pricing, technical specifications, and implementation details. Keep responses concise but informative, focusing on accurate information. Suggest booking a demo for complex requirements.",
"knowledgeBase": {
"enabled": true,
"sources": [
{
"name": "product_catalog",
"priority": 1.0,
"data": [
{
"id": "cloud-essentials",
"name": "Cloud Essentials",
"category": "Cloud Infrastructure",
"description": "Entry-level cloud computing solution for small businesses and startups.",
"features": ["2 vCPUs", "4GB RAM", "100GB Storage", "99.9% Uptime SLA"],
"priceMonthly": 49.99,
"priceAnnual": 539.99,
"discounts": {
"nonprofit": 15,
"education": 20,
"startups": 10
},
"technicalSpecs": {
"datacenterRegions": ["us-east", "us-west", "eu-central"],
"backupFrequency": "Daily",
"scalability": "Manual scaling",
"supportLevel": "Standard (24/7 email)"
}
},
{
"id": "cloud-professional",
"name": "Cloud Professional",
"category": "Cloud Infrastructure",
"description": "Professional-grade cloud solution for growing businesses with moderate workloads.",
"features": ["4 vCPUs", "16GB RAM", "500GB Storage", "99.95% Uptime SLA", "Load Balancing"],
"priceMonthly": 149.99,
"priceAnnual": 1619.99,
"discounts": {
"nonprofit": 15,
"education": 20,
"startups": 10
},
"technicalSpecs": {
"datacenterRegions": ["us-east", "us-west", "eu-central", "eu-west", "asia-east"],
"backupFrequency": "Twice daily",
"scalability": "Auto-scaling available",
"supportLevel": "Enhanced (24/7 email & phone)"
}
},
{
"id": "cloud-enterprise",
"name": "Cloud Enterprise",
"category": "Cloud Infrastructure",
"description": "Enterprise-grade cloud solution for businesses with mission-critical applications and high-demand workloads.",
"features": ["16+ vCPUs", "64GB+ RAM", "2TB+ Storage", "99.99% Uptime SLA", "Load Balancing", "Dedicated Support", "Advanced Security"],
"priceMonthly": "Custom",
"priceAnnual": "Custom",
"technicalSpecs": {
"datacenterRegions": ["All global regions"],
"backupFrequency": "Continuous",
"scalability": "Predictive auto-scaling",
"supportLevel": "Premium (24/7 dedicated TAM)"
}
}
]
},
{
"name": "implementation_process",
"priority": 0.9,
"data": {
"stages": [
{
"name": "Discovery",
"description": "In-depth assessment of your current infrastructure and requirements.",
"deliverables": ["Requirements Document", "Current State Analysis"],
"duration": "1-2 weeks"
},
{
"name": "Solution Design",
"description": "Custom architecture and implementation plan tailored to your needs.",
"deliverables": ["Solution Architecture", "Implementation Roadmap", "Resource Planning"],
"duration": "2-3 weeks"
},
{
"name": "Implementation",
"description": "Deployment of infrastructure, configuration, and migration.",
"deliverables": ["Deployed Infrastructure", "Integration Tests", "Security Validation"],
"duration": "3-8 weeks"
},
{
"name": "Training & Handover",
"description": "Comprehensive training for your team and knowledge transfer.",
"deliverables": ["Training Sessions", "Documentation", "Support Handover"],
"duration": "1-2 weeks"
},
{
"name": "Ongoing Support",
"description": "Continued assistance and optimization based on your support tier.",
"deliverables": ["Regular Reviews", "Performance Reports", "Optimization Recommendations"],
"duration": "Ongoing"
}
],
"implementationTeam": [
{
"role": "Solution Architect",
"responsibilities": "Overall solution design and technical guidance"
},
{
"role": "Project Manager",
"responsibilities": "Timeline, resources, and deliverable management"
},
{
"role": "Cloud Engineers",
"responsibilities": "Infrastructure deployment and configuration"
},
{
"role": "Migration Specialist",
"responsibilities": "Data and application migration planning and execution"
},
{
"role": "Training Specialist",
"responsibilities": "Customer team enablement and knowledge transfer"
}
],
"typicalTimelines": {
"small": "6-8 weeks total",
"medium": "10-14 weeks total",
"large": "16-24 weeks total",
"enterprise": "6+ months with phased approach"
}
}
},
{
"name": "case_studies",
"priority": 0.8,
"data": [
{
"company": "HealthTech Solutions",
"industry": "Healthcare",
"challenge": "Needed HIPAA-compliant infrastructure with high availability for patient portal",
"solution": "Cloud Enterprise with dedicated security controls and geo-redundant deployment",
"results": [
"99.997% uptime over 12 months",
"40% reduction in operational costs",
"Passed all compliance audits"
],
"quote": "TechCorp's implementation team understood our compliance requirements perfectly and delivered a solution that exceeds our expectations."
},
{
"company": "RetailGiant",
"industry": "Retail",
"challenge": "E-commerce platform struggling with seasonal traffic spikes",
"solution": "Cloud Professional with auto-scaling and global CDN integration",
"results": [
"Handled 10x traffic increase during Black Friday",
"Page load times decreased by 65%",
"Conversion rate improved by 23%"
],
"quote": "After migrating to TechCorp, our platform didn't even flinch during peak season, which used to be our annual nightmare."
}
]
},
{
"name": "support_tiers",
"priority": 0.7,
"data": [
{
"tier": "Standard",
"included": "Included with all plans",
"features": [
"24/7 email support",
"8-hour response time",
"Knowledge base access",
"Community forum access"
]
},
{
"tier": "Enhanced",
"included": "Included with Professional plan",
"features": [
"All Standard features",
"24/7 phone support",
"2-hour response time",
"Monthly system review"
],
"additionalCost": "$299/month for Essentials plan"
},
{
"tier": "Premium",
"included": "Included with Enterprise plan",
"features": [
"All Enhanced features",
"Dedicated Technical Account Manager",
"15-minute response time for critical issues",
"Quarterly business review",
"Architectural guidance",
"Priority feature requests"
],
"additionalCost": "$999/month for Professional plan, not available for Essentials"
}
]
}
]
},
"intents": [
{
"name": "pricing_inquiry",
"patterns": [
"how much does it cost",
"pricing information",
"what are your prices",
"cost of cloud solutions"
],
"responses": [
"Our Cloud Essentials plan starts at $49.99/month, Cloud Professional at $149.99/month, and Cloud Enterprise has custom pricing based on your specific requirements."
],
"followupQuestions": [
"Which plan seems most aligned with your current needs?",
"Would you like me to explain what's included in each plan?"
]
},
{
"name": "implementation_process",
"patterns": [
"how does implementation work",
"onboarding process",
"how long to deploy",
"timeline for setup"
],
"responses": [
"Our implementation follows a 5-stage process: Discovery, Solution Design, Implementation, Training & Handover, and Ongoing Support. Total timeline typically ranges from 6 weeks for small deployments to 6+ months for enterprise solutions."
],
"followupQuestions": [
"Would you like to hear more about a specific stage of the implementation process?",
"What's your expected timeline for implementation?"
]
},
{
"name": "technical_specifications",
"patterns": [
"tech specs",
"vCPU information",
"storage capacity",
"data center locations",
"uptime guarantee"
],
"responses": [
"Our solutions offer various technical specifications depending on the plan. Cloud Essentials includes 2 vCPUs/4GB RAM/100GB storage with 99.9% SLA, while Professional provides 4 vCPUs/16GB RAM/500GB storage with 99.95% SLA. Enterprise plans are fully customizable to your requirements with 99.99% SLA."
]
},
{
"name": "demo_request",
"patterns": [
"see a demo",
"schedule demonstration",
"book a tour",
"show me how it works"
],
"responses": [
"I'd be happy to arrange a personalized demo with one of our cloud specialists. They can walk you through our platform, answer specific questions, and show exactly how our solutions would work for your use case."
],
"action": "scheduleDemoWebhook"
}
],
"bookingSuggestions": {
"English": [
"Would you like to schedule a personalized demo to see our cloud solutions in action?",
"I can arrange a call with one of our technical specialists to discuss your specific requirements.",
"The best way to understand our Enterprise offering is through a custom demo - I can help schedule that.",
"Our implementation team would be happy to walk through the migration process in a personalized consultation."
]
},
"conversationFlows": [
{
"name": "pricing_to_demo",
"trigger": "intent:pricing_inquiry",
"steps": [
{
"action": "provide_pricing",
"then": "ask_requirements"
},
{
"action": "ask_requirements",
"question": "What are your primary requirements for a cloud solution?",
"then": "recommend_plan"
},
{
"action": "recommend_plan",
"then": "suggest_demo"
},
{
"action": "suggest_demo",
"suggestion": "Based on your requirements, I'd recommend seeing our [recommended_plan] in action. Would you like to schedule a personalized demo?"
}
]
}
]
}
Business Logic Automation
Configure advanced business logic to automate customer interactions:
Intent Recognition & Responses
window.HUMANBOT_CONFIG = {
businessLogic: {
intents: [
{
name: "pricing_inquiry",
patterns: [
"how much does it cost",
"pricing information",
"what are your prices"
],
responses: [
"Our plans start at $49/month for the Basic tier, with Professional at $99/month and Enterprise custom pricing.",
"We offer several pricing tiers starting from $49/month, with discounts available for annual commitments."
],
followupQuestions: [
"Which plan seems to align with your needs?",
"Would you like me to explain what's included in each plan?"
],
entities: ["pricing_plan", "billing_cycle", "user_count"],
action: "send_pricing_webhook"
},
// More intents...
],
// Intent priorities
intentPriorities: {
sales_opportunity: 0.9,
technical_support: 0.7,
general_inquiry: 0.5
},
// Entity extraction
entities: {
email: {
patterns: [
"\\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}\\b"
],
storeAs: "user.email",
validation: "standard_email"
},
phone: {
patterns: [
"\\b\\+?[0-9][0-9\\-\\s]{7,}[0-9]\\b"
],
storeAs: "user.phone",
validation: "phone_number"
},
company_size: {
patterns: [
"\\b([0-9]+)\\s*(employees|people|staff)\\b",
"company.*(size|employees).*([0-9]+)"
],
storeAs: "user.companySize",
validation: "integer"
}
// More entities...
}
}
};
Conditions & Actions
window.HUMANBOT_CONFIG = {
businessLogic: {
// ... other settings ...
conditions: [
{
name: "high_value_prospect",
rules: [
{
field: "entities.company_size",
operator: "greaterThan",
value: 100
},
{
field: "entities.budget",
operator: "greaterThan",
value: 10000
}
],
operator: "AND"
},
{
name: "ready_for_demo",
rules: [
{
field: "conversation.messageCount",
operator: "greaterThan",
value: 4
},
{
field: "entities.email",
operator: "exists"
},
{
field: "sentiment.average",
operator: "greaterThan",
value: 0.2
}
],
operator: "AND"
}
],
actions: [
{
name: "priority_routing",
trigger: "condition:high_value_prospect",
execute: [
{
type: "webhook",
endpoint: "priorityLeadAlert",
data: {
email: "{{entities.email}}",
companySize: "{{entities.company_size}}",
budget: "{{entities.budget}}",
transcript: "{{conversation.messages}}"
}
},
{
type: "update_crm",
provider: "salesforce",
action: "update_lead",
data: {
status: "Hot Lead",
priority: "High",
assignTo: "enterprise_team"
}
},
{
type: "send_email",
template: "vip_lead_notification",
to: ["sales.director@company.com", "account.exec@company.com"],
data: {
leadName: "{{entities.name}}",
companyName: "{{entities.company}}",
leadSummary: "{{conversation.summary}}"
}
}
]
},
{
name: "suggest_demo",
trigger: "condition:ready_for_demo",
execute: [
{
type: "send_message",
message: "Based on what you've shared, I think a personalized demo would be the best next step. Would you like me to schedule one with our product team?"
},
{
type: "show_calendar",
calendarType: "demo_booking",
prefill: {
name: "{{entities.name}}",
email: "{{entities.email}}",
company: "{{entities.company}}",
requirements: "{{conversation.summary}}"
}
}
]
}
]
}
};
Conversation Flows
Design guided conversation paths for specific business objectives:
Guided Flows
window.HUMANBOT_CONFIG = {
businessLogic: {
// ... other settings ...
conversationFlows: [
{
name: "lead_qualification",
trigger: "intent:pricing_inquiry",
steps: [
{
id: "ask_company",
message: "Great! I'd be happy to share our pricing. First, could you tell me a bit about your company?",
expect: "company_information",
extraction: ["company_name", "company_size", "industry"],
fallback: "No problem. To provide the most relevant pricing, could you share what industry you're in?",
next: "ask_requirements"
},
{
id: "ask_requirements",
message: "Thanks for sharing. What are your primary requirements for our solution?",
expect: "requirements",
extraction: ["use_case", "features", "timeline"],
fallback: "Just to understand your needs better, are you looking for any specific features?",
next: "ask_budget"
},
{
id: "ask_budget",
message: "That helps a lot. Do you have a budget range in mind for this project?",
expect: "budget",
extraction: ["budget_range", "constraints"],
fallback: "No worries if you don't have an exact figure. Is this something you're looking to implement in the near future?",
next: "provide_recommendation"
},
{
id: "provide_recommendation",
message: "Based on what you've shared, our {{recommended_plan}} would be the best fit for your needs. It starts at {{plan_price}} and includes {{plan_features}}.",
action: "calculate_recommendation",
next: "suggest_demo"
},
{
id: "suggest_demo",
message: "Would you like to see it in action through a personalized demo?",
expect: "demo_response",
branches: {
"positive": "schedule_demo",
"negative": "provide_more_info",
"default": "provide_more_info"
}
},
{
id: "schedule_demo",
message: "Great! Let's schedule that demo. What's the best email to reach you at?",
expect: "email",
extraction: ["email"],
action: "create_demo_booking",
next: "confirmation"
},
{
id: "provide_more_info",
message: "I understand. Here's some additional information about our {{recommended_plan}} that might be helpful: {{plan_details}}",
action: "send_brochure_email",
next: "follow_up"
},
{
id: "confirmation",
message: "Perfect! I've scheduled a demo for {{demo_time}}. You'll receive a confirmation email shortly with all the details and calendar invite.",
action: "send_confirmation",
next: "end"
},
{
id: "follow_up",
message: "Is there anything specific about our solution you'd like to learn more about?",
expect: "follow_up_question",
next: "end"
},
{
id: "end",
message: "Thank you for your interest in our solution. If you have any other questions, feel free to ask anytime!",
action: "log_conversation_complete"
}
],
variables: {
recommended_plan: "",
plan_price: "",
plan_features: "",
plan_details: "",
demo_time: ""
}
}
]
}
};
Dynamic Response Templates
window.HUMANBOT_CONFIG = {
businessLogic: {
// ... other settings ...
responseTemplates: {
product_description: {
template: "{{product_name}} is our {{product_category}} solution that {{product_benefit}}. It includes {{product_features}} and is ideal for {{target_audience}}.",
variables: {
product_name: "${entity.product.name}",
product_category: "${entity.product.category}",
product_benefit: "${entity.product.benefit}",
product_features: "${entity.product.features|join(', ')}",
target_audience: "${entity.product.audience|join(' and ')}"
}
},
pricing_information: {
template: "Our {{plan_name}} plan starts at {{price}}{{billing_cycle}}. This includes {{features}} with {{support_level}} support.",
variables: {
plan_name: "${entity.plan.name}",
price: "${entity.plan.price}",
billing_cycle: "${entity.plan.billing_cycle == 'monthly' ? '/month' : '/year'}",
features: "${entity.plan.key_features|join(', ')}",
support_level: "${entity.plan.support_level}"
}
},
demo_suggestion: {
template: "{{greeting}} I'd be happy to show you how {{solution_benefit}}. {{demo_offer}}",
variables: {
greeting: "${condition(conversation.context.customerStatus == 'returning', 'Welcome back!', 'Great question!')}",
solution_benefit: "${entity.focused_solution.benefit}",
demo_offer: "${condition(entity.user.role == 'technical', 'Our technical team can walk through the implementation details with you.', 'We can demonstrate how this works for your specific use case.')}"
}
},
}
}
};
7. API Reference
Humanbot Pro exposes a comprehensive JavaScript API for programmatic control.
JavaScript API
// Initialize (if not using the auto-initialization)
window.humanbotPro.init({
// Configuration options
});
// Open the chatbot
window.humanbotPro.open();
// Close the chatbot
window.humanbotPro.close();
// Check if model is ready
const isReady = window.humanbotPro.isModelReady();
// Check if chatbot is visible
const isVisible = window.humanbotPro.isVisible();
// Reset animations
window.humanbotPro.resetAnimation();
// Clean up resources (useful when navigating away)
window.humanbotPro.cleanup();
// Send a message programmatically
window.humanbotPro.sendMessage("Hello, I'd like to know more about your service");
// Set language
window.humanbotPro.setLanguage("fr");
// Event listeners
window.addEventListener("humanbotReady", function() {
console.log("Humanbot Pro is ready");
});
window.addEventListener("humanbotMessage", function(e) {
console.log("New message:", e.detail.message);
});
Event System
Humanbot Pro provides a rich event system for integration:
Event | Description | Event Data |
---|---|---|
humanbotReady |
Fired when the chatbot is fully loaded and ready | { timestamp } |
humanbotMessage |
Fired when a message is sent or received | { message, role, timestamp } |
humanbotOpen |
Fired when the chatbot is opened | { trigger } |
humanbotClose |
Fired when the chatbot is closed | { trigger, duration } |
humanbotIntent |
Fired when an intent is detected | { intent, confidence, entities } |
humanbotAction |
Fired when a business action is executed | { action, status, result } |
humanbotError |
Fired when an error occurs | { error, context, severity } |
Server API (Premium Plans)
Advanced customers have access to a REST API for configuration and analytics:
# Authentication
curl -X POST https://api.humanbot.pro/v1/auth \
-H "Content-Type: application/json" \
-d '{"apiKey": "YOUR_API_KEY"}'
# Update configuration
curl -X PUT https://api.humanbot.pro/v1/config \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_ACCESS_TOKEN" \
-d '{
"systemMessage": "Updated system message",
"language": "fr"
}'
# Get conversation analytics
curl -X GET https://api.humanbot.pro/v1/analytics \
-H "Authorization: Bearer YOUR_ACCESS_TOKEN"
Authentication Endpoints
Endpoint | Method | Description |
---|---|---|
/v1/auth |
POST | Obtain access token using API key |
/v1/auth/refresh |
POST | Refresh access token |
/v1/auth/revoke |
POST | Revoke access token |
Configuration Endpoints
Endpoint | Method | Description |
---|---|---|
/v1/config |
GET | Get current configuration |
/v1/config |
PUT | Update configuration |
/v1/config/knowledge |
PUT | Update knowledge base |
/v1/config/intents |
PUT | Update intent definitions |
Analytics Endpoints
Endpoint | Method | Description |
---|---|---|
/v1/analytics |
GET | Get general analytics |
/v1/analytics/conversations |
GET | Get conversation analytics |
/v1/analytics/intents |
GET | Get intent detection analytics |
/v1/analytics/users |
GET | Get user interaction analytics |
8. Performance Optimization
Humanbot Pro implements numerous performance optimizations to ensure minimal impact on your website:
Resource Loading
- Asynchronous Loading: Main script loads asynchronously without blocking page rendering
- Lazy Loading: 3D resources only load when the chatbot is opened
- CDN Distribution: Global CDN ensures fast load times worldwide
- Resource Caching: Intelligent caching of 3D model and textures
- Compressed Assets: All assets are compressed for minimal bandwidth usage
Memory Management
- Garbage Collection: Optimized memory cleanup with explicit disposal
- Texture Management: Dynamic texture quality adjustment
- Animation Optimization: Efficient animation handling with batch processing
- State Cleanup: Complete resource disposal when not in use
Rendering Optimization
- FPS Limiting: Adaptive frame rate limiting (30fps standard, 15fps low power)
- Low Power Mode: Automatic power saving when inactive
- WebGL Optimization: Efficient shader usage and scene optimization
- Visibility-based Rendering: Pauses rendering when tab is not visible
Network Optimization
- Minimal API Calls: Batched and throttled API requests
- Efficient Data Transfer: Minimized payload sizes for all communications
- Connection-aware Adaptation: Quality adjustments based on network conditions
- Error Recovery: Graceful degradation and recovery from network issues
Advanced Optimization Settings
window.HUMANBOT_CONFIG = {
performance: {
// Resource loading
preloadMode: "minimal", // Options: minimal, standard, aggressive
preloadAssets: ["core", "ui"], // Which assets to preload
lazyLoadModel: true, // Load 3D model only when needed
cacheStrategy: "session", // Options: none, session, persistent
// Memory management
disposeUnusedResources: true, // Clean up unused resources
textureQuality: "adaptive", // Options: low, medium, high, adaptive
maxTextureSize: 1024, // Maximum texture size
disposeTimeout: 30000, // Time before cleanup after closing
// Rendering optimization
standardFPS: 30, // Standard frame rate
lowPowerFPS: 15, // Low power mode frame rate
idleTimeout: 15000, // Timeout before entering low power mode
adaptiveQuality: true, // Adjust quality based on device
prioritizePerformance: true, // Favor performance over visual quality
// Network optimization
minRequestInterval: 500, // Minimum ms between API calls
batchRequests: true, // Batch API requests
compressionLevel: "high", // Data compression level
retryStrategy: "exponential", // How to retry failed requests
offlineSupport: true // Enable offline capabilities
}
};
9. Use Cases
E-commerce & Retail
window.HUMANBOT_CONFIG = {
systemMessage: "You are a helpful shopping assistant. Help customers find products, answer questions about shipping, returns, and provide personalized recommendations. Keep responses short and friendly.",
productHighlights: {
"English": [
"Free shipping on orders over $50",
"30-day money-back guarantee",
"24/7 customer support"
]
}
};
Benefits:
- Reduce cart abandonment by answering questions immediately
- Guide customers to relevant products through natural conversation
- Provide instant support for order status and return questions
- Collect customer preferences for better recommendations
SaaS Companies
window.HUMANBOT_CONFIG = {
systemMessage: "You are a product specialist for our SaaS platform. Help visitors understand our features, pricing, and how our solution solves their problems. Suggest booking a demo for detailed questions.",
bookingSuggestions: {
"English": [
"Would you like to see how this works in a quick demo?",
"I can set up a demo call with our product team if you'd like to see this in action."
]
}
};
Benefits:
- Qualify leads through natural conversation
- Increase demo bookings with timely suggestions
- Provide instant answers to common questions
- Reduce sales team workload for basic inquiries
Real Estate
window.HUMANBOT_CONFIG = {
systemMessage: "You are a real estate assistant. Help potential buyers and renters understand property features, neighborhood information, and financing options. Suggest property viewings for interested prospects.",
personalityTraits: {
"knowledgeable": "Share detailed insights about properties and neighborhoods",
"helpful": "Guide prospects through the buying or renting process",
"professional": "Maintain a professional tone while being approachable"
}
};
Benefits:
- Qualify property inquiries before agent involvement
- Provide 24/7 property information to international prospects
- Schedule viewings through natural conversation
- Answer detailed questions about neighborhoods and amenities
Healthcare Providers
window.HUMANBOT_CONFIG = {
systemMessage: "You are a healthcare information assistant. Help patients find the right department, understand services, and book appointments. Do not provide medical advice. Maintain HIPAA compliance by never asking for or storing personal health information.",
workingHours: {
weekdays: "8:00-17:00",
weekend: "Closed",
timezone: "America/New_York",
offHoursMessage: "Our office is currently closed. For medical emergencies, please call 911. For non-emergencies, leave a message and we'll respond during business hours."
}
};
Benefits:
- Direct patients to appropriate departments
- Reduce administrative call volume for appointment scheduling
- Provide instant answers about services, insurance, and preparation
- Maintain compliance with healthcare regulations
Financial Services
window.HUMANBOT_CONFIG = {
systemMessage: "You are a financial services assistant. Help visitors learn about investment options, account services, and financial planning. For specific account questions, suggest speaking with a financial advisor.",
bookingSuggestions: {
"English": [
"Would you like to schedule a consultation with one of our financial advisors?",
"Our financial advisors are available for personalized discussions. Would you like to book a call?"
]
},
security: {
piiDetection: true,
dataRetentionPeriod: 0,
encryptionEnabled: true
}
};
Benefits:
- Educate prospects on complex financial products
- Schedule advisor consultations efficiently
- Provide 24/7 support for basic account questions
- Maintain compliance with financial regulations
10. Browser Compatibility
Humanbot Pro is designed to work across all modern browsers with WebGL support.
Desktop Browsers
- Chrome 69+
- Firefox 63+
- Safari 12+
- Edge 79+ (Chromium-based)
- Opera 56+
Mobile Browsers
- iOS Safari 12+
- Android Chrome 69+
- Samsung Internet 10+
- Android Firefox 63+
Requirements
- WebGL 1.0 support (WebGL 2.0 recommended for optimal performance)
- JavaScript enabled
- Cookies enabled for session persistence
- Minimum 2GB RAM (4GB recommended)
- Modern CPU (released after 2017)
Performance Notes
- Low-End Devices: Automatically adjusts quality and framerate
- Older Browsers: Falls back to simplified UI without 3D
- No WebGL: Provides text-only chat functionality
- Slow Connections: Adaptive loading with reduced assets
Browser Feature Detection
window.HUMANBOT_CONFIG = {
compatibility: {
// Browser detection
fallbackForOlderBrowsers: true, // Enable fallback for older browsers
textOnlyFallback: true, // Enable text-only fallback for no WebGL
mobileOptimizations: true, // Enable mobile-specific optimizations
// Required features
requiredFeatures: {
webgl: "fallback", // Options: required, recommended, fallback
webSpeech: "recommended", // Options: required, recommended, fallback
localStorage: "recommended", // Options: required, recommended, fallback
cookies: "fallback", // Options: required, recommended, fallback
modernJavascript: "required" // Options: required, recommended, fallback
},
// Custom detection and fallbacks
customChecks: [
{
feature: "webpSupport",
test: "function() { return !!(document.createElement('canvas').toDataURL('image/webp').indexOf('data:image/webp') === 0); }",
fallback: "function() { window.HUMANBOT_CONFIG.performance.imageFormat = 'png'; }"
},
{
feature: "touchSupport",
test: "function() { return 'ontouchstart' in window || navigator.maxTouchPoints > 0; }",
fallback: "function() { /* No action needed */ }"
}
]
}
};
11. Troubleshooting
Common Issues
Installation Issues
Issue: Script fails to load
Solution: Verify the script URL is correct and your network allows the connection. Check browser console for specific errors.
// Add error handling to script loading
const script = document.createElement('script');
script.src = "https://cdn.humanbot.pro/embed.min.js";
script.async = true;
script.onerror = function() {
console.error("Failed to load Humanbot Pro script");
};
document.head.appendChild(script);
Issue: 3D model doesn't appear
Solution: Ensure WebGL is supported and enabled in the browser. Try forcing software rendering.
window.HUMANBOT_CONFIG = {
rendering: {
forceSoftwareRendering: true,
fallbackToSimpleUI: true
}
};
Performance Issues
Issue: High CPU usage
Solution: Adjust performance settings to reduce resource usage.
window.HUMANBOT_CONFIG = {
performance: {
limitFPS: 20,
lowPowerTimeout: 10000,
reduceAnimationQuality: true,
disableBackgroundEffects: true
}
};
Issue: Memory leaks
Solution: Ensure cleanup is called when navigating between pages in SPAs.
// React example in componentWillUnmount
componentWillUnmount() {
if (window.humanbotPro && window.humanbotPro.cleanup) {
window.humanbotPro.cleanup();
}
}
API Related Issues
Issue: No responses from the chatbot
Solution: Verify API key and network connections.
window.HUMANBOT_CONFIG = {
debug: true,
logLevel: "verbose",
fallbackResponses: [
"I'm having trouble connecting right now. Please try again in a moment.",
"It seems our services are temporarily unavailable. Please check back soon."
]
};
Diagnostic Tools
-
Built-in Diagnostics
JavaScript
window.humanbotPro.runDiagnostics().then(results => { console.log("Diagnostics:", results); });
-
Performance Monitoring
JavaScript
window.humanbotPro.startPerformanceMonitoring(); // Later const metrics = window.humanbotPro.getPerformanceMetrics(); console.log("Performance:", metrics);
-
Debug Mode
JavaScript
window.HUMANBOT_CONFIG = { debug: true, logLevel: "verbose" // Options: error, warn, info, verbose, debug };
Common Error Codes
Error Code | Description | Solution |
---|---|---|
HBP-001 |
API Authentication Failed | Verify API key is correct and has not expired |
HBP-002 |
WebGL Not Supported | Enable WebGL in browser or use fallback mode |
HBP-003 |
3D Model Load Failed | Check network connection or use simplified UI |
HBP-004 |
API Rate Limit Exceeded | Reduce request frequency or upgrade plan |
HBP-005 |
Configuration Invalid | Check configuration syntax and required fields |
12. Technical FAQs
General Questions
Q: How much does Humanbot Pro impact my website's performance?
A: Humanbot Pro is designed to have minimal impact on your website. The initial load is approximately 5MB, with assets loaded progressively and only when needed. The 3D rendering uses optimized shaders and texture management to reduce GPU usage, and the system automatically scales down quality on lower-end devices.
Q: Is Humanbot Pro GDPR compliant?
A: Yes, Humanbot Pro is designed with privacy in mind. All data processing happens through your account's API credentials, and no personal data is stored by default. The system can be configured to automatically detect and redact PII (Personally Identifiable Information) in conversations.
Q: How do I integrate Humanbot Pro with my CRM?
A: Humanbot Pro offers webhooks for major CRM systems including Salesforce, HubSpot, and Zoho. Conversations can be automatically logged, and lead information can be passed directly to your CRM. Custom integrations are available through our REST API.
Development Questions
Q: Can I customize the 3D avatar?
A: Yes, enterprise plans include options for custom avatars. You can provide your own glTF/GLB model with specific requirements for bone structure and morph targets. Our team can assist with converting and optimizing your custom model.
Q: How do I implement Humanbot Pro in a React/Angular/Vue application?
A: We provide official packages for major frameworks:
- React:
@humanbot/react
- Angular:
@humanbot/angular
- Vue:
@humanbot/vue
These packages offer framework-specific implementations with proper lifecycle management.
Q: Can Humanbot Pro access my website's content to answer questions?
A: Yes, with the Knowledge Base feature (premium plans), Humanbot Pro can be trained on your website content, documentation, and FAQs. This allows the assistant to provide accurate, website-specific information to visitors.
Language & Voice Questions
Q: Which languages are supported?
A: Humanbot Pro supports 72+ languages for text interactions. For voice capabilities, we support 30+ languages with realistic text-to-speech through ElevenLabs integration.
Q: Can I use my own voice for the assistant?
A: Yes, enterprise plans support custom voice cloning through our ElevenLabs integration. This requires approximately 5 minutes of clear voice recordings and our team will assist with the setup process.
Q: How accurate is the speech recognition?
A: Speech recognition uses the browser's native Web Speech API enhanced with our noise reduction algorithms. Recognition accuracy varies by language and environment, but typically achieves 90%+ accuracy in standard conditions with clear speech.
Security Questions
Q: Where is my API key stored?
A: Your API key is never stored in the browser. All API calls are proxied through our secure backend to protect your credentials. This architecture ensures your API keys remain secure even if someone inspects your website's code.
Q: Does Humanbot Pro store conversation data?
A: By default, Humanbot Pro stores conversation data for 30 days to provide context for returning visitors. This can be configured to your requirements, including zero retention if needed for compliance purposes.
Q: Is communication encrypted?
A: Yes, all communication between the browser and our servers uses TLS 1.3 encryption. API keys and sensitive data are additionally encrypted at rest using AES-256.